posted at 2022.10.1 03:04 by Administrator
一、系统需求:
停车场能够停放 n 辆车,车按到达的先后次序停放,车辆可以通过车牌区分。实时了解停车场利用率,利用率为100%时,车要在门外的便道上等候。停车场中的车通过公共车道离开,车辆按停放时间收费。
二、基本要求:
1)建立栈(Stack),实现数据存储。
2)模拟车辆出入停车场过程。
3)车辆按停放时间收费。
三、Go源代码:
package main import ( "fmt" "sync" "time") const Stack_Size int = 5const cash float64 = 0.00005 type car struct { number string carCent float64 seconds int64}type stackA struct { //停车场栈 carNode [Stack_Size]car top int lock sync.Mutex // 为了并发安全使用的锁}type stackB struct { //临时栈 carNode [Stack_Size]car top int lock sync.Mutex // 为了并发安全使用的锁} func initStackA(parkingStack *stackA) { parkingStack.top = -1}func initStackB(tempStack *stackB) { tempStack.top = -1}func listStack(pl *stackA) { if pl.top != -1 { var index int fmt.Printf("\n序列号\t车牌号\t停车场利用率\t进场时间") for index = 0; index <= pl.top; index++ { tm := time.Unix(pl.carNode[index].seconds, 0) fmt.Printf("\n%v\t%s\t%.2f\t%v", index+1, pl.carNode[index].number, pl.carNode[index].carCent, tm) } } else { fmt.Println("\n目前没有停车!") }}func backPL(pl *stackA, ts *stackB) { //将临时栈的车开回停车场,更新车道值 pl.top = 0 for ts.top != -1 { //当临时栈不为空栈 pl.carNode[pl.top] = ts.carNode[ts.top] pl.top++ ts.top-- }}func arrival(pl *stackA, st *stackB) { p := time.Now() t1 := p.Unix() var num string fmt.Printf("\n请输入车牌号:\n") fmt.Scanln(&num) if pl.top == Stack_Size-1 { //当车位已满 fmt.Printf("\n车位已满,%s号车辆进入便道等候!", num) } else { //当车位未满 pl.top++ pl.carNode[pl.top].seconds = t1 pl.carNode[pl.top].number = num pl.carNode[pl.top].carCent = float64((pl.top + 1)) / float64(Stack_Size) fmt.Printf("\n停车成功!") }}func leave(pl *stackA, ts *stackB) { var index int p := time.Now() t2 := p.Unix() listStack(pl) fmt.Printf("\n请输入要出场车辆的序列号:") fmt.Scanf("%d", &index) sum := float64(pl.top) / float64(Stack_Size) if index-1 > pl.top || index-1 < 0 { //index-1对应pl->top fmt.Printf("\n请输入正确的序列号!") fmt.Printf("\n按任意键继续!") } else { ts.top = -1 for pl.top != -1 { //当要出车的序列号不等于栈顶时,进临时栈 if pl.top == index-1 { pl.top-- continue } ts.top++ ts.carNode[ts.top] = pl.carNode[pl.top] pl.top-- } //此时要出车的车道等于栈顶时,直接出车,不需进临时栈 fmt.Printf("\n提车成功!") fmt.Printf("\n%s的驶离时间为%v", pl.carNode[index-1].number, p) fmt.Printf("\n停车收费合计 %2.1f元", float64(t2-pl.carNode[index-1].seconds)*cash) fmt.Printf("\n此时停车场利用率为%.2f", sum) backPL(pl, ts) pl.top-- //清除已出车数据 }}func menu() int { var flag int = 0 fmt.Printf("\n************欢迎使用停车场管理系统************") fmt.Printf("\n 按[1]停车") fmt.Printf("\n 按[2]提车") fmt.Printf("\n 按[3]查看停车场状况") fmt.Printf("\n 按[4]退出系统") fmt.Printf("\n***************************************\n") if flag < 1 || flag > 4 { fmt.Printf("请输入1~4!\n") } fmt.Scanln(&flag) return flag}func quit() { fmt.Printf("\n\n谢谢使用!") return}func main() { var pl stackA var ts stackB initStackA(&pl) initStackB(&ts)timedy: switch menu() { case 1: arrival(&pl, &ts) goto timedy case 2: leave(&pl, &ts) goto timedy case 3: listStack(&pl) goto timedy case 4: quit() default: goto timedy }}
四、部分效果图:
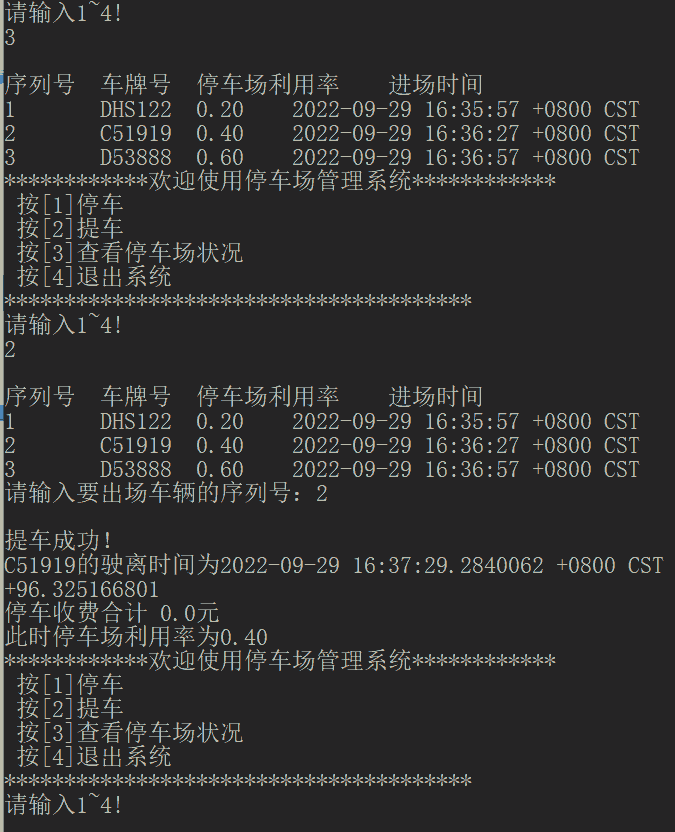
fb624c09-2343-4fcc-92eb-05d7807b7974|0|.0|96d5b379-7e1d-4dac-a6ba-1e50db561b04
Tags: 车, 代码, 模, 数据
IT技术